前端–jQuery滚动事件
scroll() 滚动条事件
scrollLeft()
获取的是横向滚动的距离。
scrollTop()
获取的纵向滚动的距离。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq滚动事件</title> <style> * { margin: 0; padding: 0; }
ul, li { list-style: none; }
#box { width: 2000px; height: 3000px; background-color: #dbdbdb; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script>
$(function () { $(window).scroll(function () { console.log($(window).scrollTop()); console.log($(this).scrollLeft()); }) });
</script> </head> <body> <div id="box">喵瞄</div> </body> </html>
|
1. 滚动事件实现广告跟随
广告跟随: 当前图片的位置= 滚动滚动的距离 + 当前图片与浏览器上边框的距离。
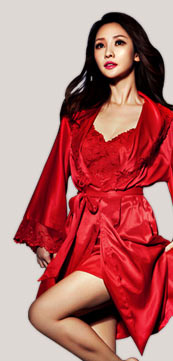
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>广告跟随</title> <style> * { margin: 0; padding: 0; } ul, li { list-style: none; } #box { height: 3000px; } img{ position:absolute; left:0px; top:0px; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script>
$(function () { $(window).scroll(function(){ // 广告跟随: 当前图片的位置= 滚动滚动的距离 + 当前图片与浏览器上边框的距离。 var top1 = $(this).scrollTop(); $("img").stop().animate({"top":(top1+160)+"px"},2000); }) }); </script> </head> <body> <img src="../images/03.jpg" alt=""> <div id="box"></div> </body> </html>
|
2. 小火箭返回最顶端
$(window).height();
获取的是当前浏览器可视区域的高度。
$(window).scrollTop();
滚动条滚动的距离纵向。


1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>小火箭返回最顶端</title> <style> * { margin: 0; padding: 0; }
ul, li { list-style: none; }
body { height: 3000px; }
#box { height: 900px; background-color: #dad8d8; }
img { position: fixed; right: 0px; bottom: 0px; display: none; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> /** * $(window).height();获取的是当前浏览器可视区域的高度。 * $(window).scrollTop();滚动条滚动的距离纵向 */ $(function () { $(window).scroll(function () { console.log(this); if ($(window).scrollTop() >= $(window).height()) { $("img").slideDown(200); } else { $("img").slideUp(200); } }); $("img").mouseenter(function () { $(this).prop("src", "../images/02.gif"); }).mouseleave(function () { $(this).prop("src", "../images/01.png"); }).click(function () { /** * $("html") 在谷歌浏览器生效了 * $("body") 在火狐浏览器生效。 */ $("body,html").stop().animate({"scrollTop": "0px"}, 500); }) }); </script> </head> <body> <div id="box"></div> <img src="../images/01.png" alt=""> </body> </html>
|
3. 电梯导航
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152
| <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>itbuild.cn</title> <style type="text/css"> * { margin: 0; padding: 0; list-style: none; }
.header { width: 1000px; height: 600px; margin: 0 auto 50px; font-size: 60px; font-weight: 700; }
.main { width: 1000px; margin: 0 auto 50px; font-size: 60px; font-weight: 700; }
.main div { height: 600px; margin: 0 auto 50px; }
.nav { width: 100%; height: 50px; background: rgba(0, 0, 0, 0.5); position: fixed; left: 0; top: 0; display: none; }
.nav ul { width: 1200px; height: 50px; margin: 0 auto; }
.nav ul li { float: left; }
.nav ul li.current { background: #6495ED; }
.nav ul li a { display: block; width: 120px; height: 50px; line-height: 50px; text-align: center; color: #fff; text-decoration: none; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> $(window).scroll(function () { if ($(this).scrollTop() >= 600) { $(".nav").slideDown(200); } else { $(".nav").slideUp(200); } $(".nav ul li").each(function (index, ele) { if ($(window).scrollTop() >= $(".main div").eq(index).offset().top - 50) { $(this).addClass("current").siblings().removeClass("current"); } }); $(".nav ul").on("click", "li", function () { var index1 = $(this).index(); var top1 = $(".main div").eq(index1).offset().top; $("html,body").stop().animate({"scrollTop": (top1 - 50) + "px"}, 1000); }) }) </script>
</head>
<body> <div class="nav"> <ul> <li> <a href="##">JavaEE</a> </li> <li> <a href="##">Android</a> </li> <li> <a href="##">PHP</a> </li> <li> <a href="##">UI设计</a> </li> <li> <a href="##">iOS</a> </li> <li> <a href="##">前段与移动开发</a> </li> <li> <a href="##">C/C++</a> </li> <li> <a href="##">网络营销</a> </li> <li> <a href="##">Python</a> </li> <li> <a href="##" style="border:0 none">云计算之大数据</a> </li>
</ul> </div> <div class="header" style="background: red;">头部</div> <div class="main"> <div class="java" style="background:#0099CC;">java</div> <div class="android" style="background:#A4FF34;">android</div> <div class="php" style="background:green;">php</div> <div class="ui" style="background:blue;">ui</div> <div class="ios" style="background:blueviolet;">ios</div> <div class="h5" style="background:gray;">h5</div> <div class="c" style="background:yellow;">c</div> <div class="net" style="background:black;">net</div> <div class="python" style="background:cadetblue;">python</div> <div class="cal" style="background:darkblue;">cal</div>
</div> </body>
</html>
|
☆