[toc]
前端–JQuery入门
1. jQuery简介
- JavaScript 在1995年时,由Netscape公司的Brendan Eich,在网景导航者浏览器上首次设计实现而成。jQuey是一个快速、简洁的JavaScript框架,在2006年1月由
John Resig
等人创建。
- jQuery的作用:因为以后的工作中,会有一些
老项目
,大部分都在使用jQuery,jQuery是前端程序员必须会的知识。jQuery可以帮助我们更好的理解JavaScript
。
- jQuery解决了95%的JavaScript兼容问题。我们程序员几乎不用操心兼容问题了,将经历放到具体的业务逻辑上就可以。
2. jQuery的版本
源码版本:
方便我们来读和学习里面的代码。
压缩版:
.min.js 项目上线的时候使用。
1 2 3 4 5
| 1.xxx版本.js
2.xxx版本.js
3.xxx版本.js
|
- 点击下载jQuery的各个版本…
- 当然也可以使用jQuery CDN,使用时要确保网络通畅哦!
- 我们则使用
jquery-1.10.1.js
这个版本进行学习。
3. jQuery的优点
- 使用简单方便:
宗旨:write less ,do more
- jQuery能做的事情,JS都能做。
- jQuery简单易懂,兼容性好。
- 链式编程
- 式编程
4. jQuery初体验
- jQuery都是用
$
开头的,$("选择器")
==事件==是一个函数,==事件处理函数通过事件的参数传递。==
this是JavaScript里面的对象,$(this) 是jQuery的对象。
- hide是隐藏,如果加了时间,会有动画效果。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jQuery初体验</title> <script src="js/jquery-1.10.1.js"></script> <style> .box { width: 200px; height: 200px; background-color: red; } </style> </head> <body> <button class="box"></button> <button class="box"></button> </body> <script>
$(".box").click(function () { $(this).hide(1000); })
</script> </html>
|
4.1 js对象和jq对象之间的转换☆
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>js对象和jq对象之间的转换</title> <script> window.onload = function () { var btn = document.getElementById("btn"); console.dir(btn); console.dir($(".btn")); console.dir($); console.dir($===jQuery);
var btn1 = $(".btn")[0]; btn1.onclick = function () { alert(111); };
var newBtn2 = $(".btn").get(1); newBtn2.onclick = function () { alert(2222); } }
</script> <script src="js/jquery-1.10.1.js"></script> </head> <body> <button class="btn">按钮</button> <button class="btn">按钮</button> </body> </html>
|

4.2 js和jq页面加载事件的区别
无论JavaScript中的onload还是jQuery中的load事件
,他们的运行时机都是当文档内容完全加载完成(包括图像、脚本文件、CSS文件等)之后,才执行当前的事件处理函数。
- JavaScript中window.onload事件只会执行一个。
- 但是jQuery中因为是调用方法,方法可以多次调用,可以执行多次,后面的不会覆盖前面的。
- 但是jQuery中的
$(document).ready(
),加载完成之后就可以执行了,不需要等到图片加载完成。可以注册多个相同的事件。
- 下面页面的效果是
窗口弹出顺序为:5---->6---->7---->加载按钮---->2---->3----->4
。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>js和jq页面加载事件的区别</title> <script src="js/jquery-1.10.1.js"></script> <script> window.onload=function(){ alert(1); }; window.onload=function(){ alert(2); }; $(window).load(function(){ alert(3); });
$(window).load(function(){ alert(4); });
$(document).ready(function(){ alert(5); }); $(function(){ alert(6); }); $(function(){ alert(7); }); </script>
</head> <body> <button id="btn">按钮</button> </body> </html>
|
4.3 jq给div设置css样式
eq(index) 获取jq对象中,多个元素中的一个。
注意要与js对象和jq对象之间的转换区分开来。
单个属性设置: css("属性名","属性值");
链式编程 $("div").css("width","200px").css("height","200px").css("background-color","red");
多个属性设置:
$("选择器").css({"属性名":"属性值","属性名1":"属性值1","属性名2":"属性值2"});
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq给div设置css样式</title> <script src="js/jquery-1.10.1.js"></script> <script> $(function () {
$("button").eq(0).click(function () { $("div").css("width", "250px"); });
$("button").eq(1).click(function () { $("div").css("height", "250px"); });
$("button").eq(2).click(function () { $("div").css("background-color", "pink"); }); $("button").eq(3).click(function () { $("div").css("width", "250px").css("height", "250px").css("background-color", "pink"); });
$("button").eq(4).click(function () { var h = $("div").css("backgroundColor"); console.log(h); }) })
</script> </head> <body> <button>变宽</button> <button>变高</button> <button>变色</button> <button>三变</button> <button>读取</button> <div></div> </body> </html>
|
4.4 jq设置和读取标签的属性
设置标签属性:
- 单标签:
目标.attr("属性名","属性值");
- 链式编程:
目标.attr("属性名","属性值").attr("属性名","属性值");
- 多属性设置
目标.attr({"属性名":"属性值","属性名1":"属性值1"});
获取属性值
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jquery设置和读取标签的属性</title> <script src="js/jquery-1.10.1.js"></script> <script> $(function () { console.log("打印所有的div:"); console.log($("div"));
$("button").eq(0).click(function () { console.log("点击button之后:"); $("div").eq(0).attr("title", "你好1");
$("div").eq(1).attr("title","你好2").attr("num","222"); $("div").css("background-color","red");
console.log("点击button打印所有的div:"); console.log($("div")); console.log("jq获取元素属性值:"); var atVlue = $("div").eq(1).attr("title"); console.log(atVlue); }); }); </script> </head> <body> <button>按钮</button> <div title="1">我是一个div</div> <div title="2">我第二个div</div> </body> </html>
|
4.5 jq设置和获取标签的内容
- 设置
目标.text("文本内容");
和innerText用法一样。
目标.html("html内容");
和innerHTML 用法一样。
- 获取
目标.text();
获取html内容,不包含标签。
目标.html():
获取html内容,包含标签。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq设置和获取标签的内容</title> <script src="js/jquery-1.10.1.js"></script> <script> $(function () { $("#btn").click(function () { console.log($("#box").html()); console.log($("#box").text()); });
$("#btn1").click(function () { $("#box").text("<h2>设置div内容</h2>"); }) }) </script> </head> <body> <button id="btn">获取</button> <button id="btn1">设置</button> <div id="box"><h1>我是div内容</h1></div> </body> </html>
|
4.6 jq简单动画效果
==显示隐藏效果:==
==卷帘门效果:==
==淡入淡出效果:==
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq简单动画效果</title> <style> #box { width: 800px; height: 800px; background-color: lightgreen; } </style> <script src="js/jquery-1.10.1.js"></script> <script> $(function () {
$("button").eq(0).click(function () { $("#box").hide(2000); }); $("button").eq(1).click(function () { $("#box").show(2000); }); $("button").eq(2).click(function () { $("#box").toggle(2000); });
$("button").eq(3).click(function () { $("#box").slideUp(2000); }); $("button").eq(4).click(function () { $("#box").slideDown(2000); }); $("button").eq(5).click(function () { $("#box").slideToggle(2000); });
$("button").eq(6).click(function () { console.log(123); $("#box").fadeIn(1000); }); $("button").eq(7).click(function () { $("#box").fadeOut(1000); }); $("button").eq(8).click(function () { $("#box").fadeTo(1000, 0.4); }); $("button").eq(9).click(function () { $("#box").fadeToggle(1000); }); }) </script> </head> <body> <div> <button>hide</button> <button>show</button> <button>toggle</button> <br> <br> <button>slidUp</button> <button>slidDown</button> <button>slidToggle</button> <br> <br> <button>fadeIn</button> <button>fadeOut</button> <button>fadeTo</button> <button>fadeToggle</button> <br> <br> <div id="box"></div> </div> </body> </html>
|
4.7 jq常用选择器
css中能用的选择器在jq中都能用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq常用选择器</title> <script src="js/jquery-1.10.1.js"></script> <script> $(function () {
$("button").eq(0).click(function () { $("p").css("color", "lightgreen") }); $("button").eq(1).click(function () { $(".left").css("color", "blue") });
$("button").eq(2).click(function () { $("#box").css("color", "pink") });
$("button").eq(3).click(function () { $("*").css("color", "green") });
$("button").eq(4).click(function () { $("h1,h2,h3").css("color", "lightblue") });
$("button").eq(5).click(function () { $("ul li").css("color", "blue") });
$("button").eq(6).click(function () { $(".box>div").css("border", "10px solid pink") });
$("button").eq(7).click(function () { $("p+div").css("color", "blue"); });
$("button").eq(8).click(function () { $("p~div").css("color", "blue"); }); }) </script> </head> <body> <button>选择所有段落标签</button> <button>选择class为left的标签</button> <button>选择id为box的标签</button> <button>选择所有标签</button> <button>选择所有标题标签</button> <br> <br> <button>选择ul里面的li标签</button> <button>选择类名box盒子的第一级子盒子添加边框</button> <button>选择p后紧挨着的一个同级div</button> <button>选择p后的所有同级div</button>
<p>段落标签</p> <p>段落标签</p> <p>段落标签</p>
<div class="left">类选择器</div> <div class="left">类选择器</div>
<div id="box">id选择器</div> <h1>标题1标签</h1> <h2>标题2标签</h2> <h3>标题3标签</h3> <ul> <li>ul列表项</li> <li>ul列表项</li> <li>ul列表项</li> <div>我也是div</div> </ul> <ol> <li>ol列表项</li> <li>ol列表项</li> <li>ol列表项</li> </ol>
<div class="box"> <div> 第一级子div <div> 第二级子div <div> 第三级子div</div> </div> </div> <div>第一级子div</div> <div>第一级子div</div> </div> </body> </html>
|
4.8 jq过滤选择器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>jq过滤选择器</title> <script src="js/jquery-1.10.1.js"></script> <style> ul, li { list-style: none; } </style> <script> $(function () { $("button").eq(0).click(function () { $("li:first").css("color", "lightgreen"); });
$("button").eq(1).click(function () { $("li:last").css("color", "lightgreen"); }); $("button").eq(2).click(function () { $("li:even").css("color", "lightgreen"); });
$("button").eq(3).click(function () { $("li:odd").css("color", "lightgreen"); }); $("button").eq(4).click(function () { $("li:odd").css("background-color", "lightgreen"); $("li:even").css("background-color", "lightpink") }); $("button").eq(5).click(function () { $("li:eq(5)").css("color", "lightgreen"); }); $("button").eq(6).click(function () { $("li:gt(5)").css("color", "lightgreen"); }); $("button").eq(7).click(function () { $("li:lt(5)").css("color", "lightgreen"); });
$("button").eq(8).click(function () { $("li:not(:eq(2))").css("color", "lightgreen");
}); $("button").eq(9).click(function () { $("li:not(:last)").css("color", "pink"); }) }) </script> </head> <body> <button>选择第一个li</button> <button>选择最后一个li</button> <button>选择所有编号为偶数个li</button> <button>选择所有编号为奇数个li</button> <button>隔行变色</button> <br> <br> <button>选择下标等于第5个的li</button> <button>选择下标大于第5个的li</button> <button>选择下标小于第5个的li</button> <button>选择所有li排除下标为2的</button> <button>选择所有li排除最后一个</button>
<ul> <li>1这是一个li</li> <li>2这是一个li</li> <li>3这是一个li</li> <li>4这是一个li</li> <li>5这是一个li</li> <li>6这是一个li</li> <li>7这是一个li</li> <li>8这是一个li</li> <li>9这是一个li</li> <li>10这是一个li</li> </ul> </body> </html>
|
5. jQuery练习案例
5.1 照相机品牌列表


1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>照相机品牌列表</title> <style> * { margin: 0; padding: 0; }
#box { width: 600px; padding: 10px; margin: 100px auto 0px; }
ul, li { list-style: none; }
.clearfix:after { content: ""; display: block; clear: both; }
#box { border: 2px solid #ccc; }
#box > ul > li { float: left; width: 200px; text-align: center; height: 25px; line-height: 25px; }
#box > .showAll { width: 200px; text-align: center; margin: 20px auto; height: 25px; line-height: 25px; border: 1px solid #ccc; cursor: pointer; background: url(../img/down.gif) 20px center no-repeat; }
.title { width: 200px; text-align: center; margin: 20px auto; height: 25px; line-height: 25px; cursor: pointer; } </style> <script src="js/jquery-1.10.1.js"></script> <script> $(function () { var flag = true; var str = ""; $("#box>ul>li:lt(12):gt(2)").hide();
$(".showAll").click(function () { flag = !flag; if (flag) { $(this).css("backgroundImage", "url(../img/down.gif)"); str = "显示所有内容"; } else { $(this).css("backgroundImage", "url(../img/up.gif)"); str = "隐藏所有内容"; } $(this).text(str); $("#box>ul>li:gt(2):not(:last)").slideToggle(2000); });
}) </script> </head> <body> <div id="box"> <div class="title">照相机品牌列表</div> <ul class="clearfix"> <li>佳能</li> <li>索尼</li> <li>三星</li> <li>尼康</li> <li>松下</li> <li>卡西欧</li> <li>富士</li> <li>柯达</li> <li>宾得</li> <li>理光</li> <li>奥林巴斯</li> <li>明基</li> <li>其他品牌相机</li> </ul> <div class="showAll">显示所有内容</div> </div>
</body> </html>
|
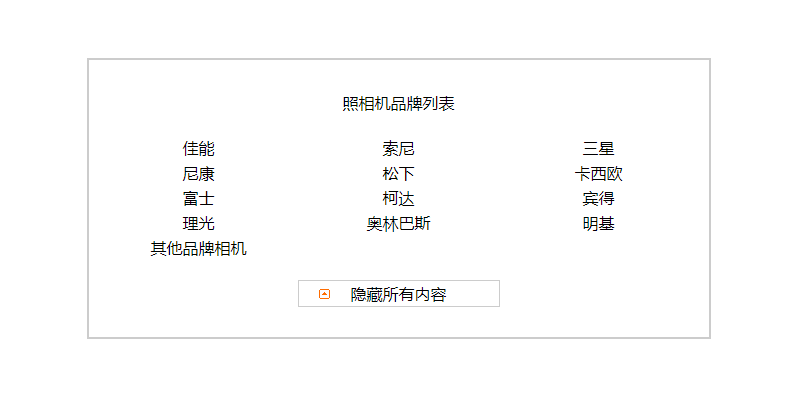
5.2 对联广告
注意本案例使用的是jQuery CDN ,测试请确保网络畅通。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>对联广告</title> <style> * { margin: 0; padding: 0; }
p { height: 40px; }
div { width: 100px; height: 300px; background-color: lightgreen; position: fixed; top: 0px; }
#box1 { left: 0px; }
#box2 { right: 0px; }
div > span { display: block; width: 20px; height: 20px; background-color: pink; text-align: center; line-height: 20px; position: absolute; cursor: pointer; }
#box1 > span { right: 0px; }
#box2 > span { left: 0px; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> $(function () { $("div>span").click(function () { $(this).parent("div").hide(500); }) }) </script> </head> <body> <div id="box1"> <span>×</span> </div> <div id="box2"> <span>×</span> </div> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> <p>段落</p> </body> </html>
|
鼠标划入事件
1 2 3
| $("div").mouseenter(function(){ })
|
5.3 轮播图导航圈排他思想
addClass()
给dom对象添加一个class样式。
removeClass()
给dom对象删除一个class样式。
siblings()
当前dom对象的所有的兄弟元素。
attr()
添加属性。
removeAttr()
移除属性。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>轮播图导航圈排他思想</title> <style> *{ margin: 0; padding: 0; } ul,li{ list-style:none; } .clearfix:after{ content:""; display:none; clear:both; } ul>li{ float:left; border: 1px solid #ccc; border-radius:50%; height:20px; width:20px; cursor:pointer; } ul>.current{ background-color: lightgreen; }
</style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> $(function(){ $("ul>li").click(function(){ $(this).addClass("current").siblings().removeClass("current"); }) })
</script> </head> <body> <ul> <li class="current"></li> <li></li> <li></li> <li></li> <li></li> </ul> </body> </html>
|
5.4 自定义单选和多选按钮
hasClass("current")
判断一个dom对象是否拥有current,如果有,返回true,否则返回false。
toggleClass("current")
判断一个对象是否拥有current样式,如果有,就删除,如果没有就添加。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>自定义单选和多选按钮</title> <style> * { padding: 0; margin: 0; }
ul, li { list-style: none; }
#radio > li { background: url(../img/radio.gif) 0 0 no-repeat; height: 18px; padding-left: 20px; cursor: pointer; }
#radio > .current { background: url(../img/radio.gif) 0 -16px no-repeat; }
#checkbox > li { background: url(../img/duo1.png) 0 0 no-repeat; height: 18px; padding-left: 20px; cursor: pointer; }
#checkbox > .current { background: url(../img/duo2.png) 0 0 no-repeat; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> $(function () { $("#radio>li").click(function () { $(this).addClass("current").siblings().removeClass("current"); })
$("#checkbox>li").click(function () { $(this).toggleClass("current"); })
}) </script> </head> <body> <ul id="radio"> <li>我是一个单选框</li> <li>我是一个单选框</li> <li>我是一个单选框</li> <li>我是一个单选框</li> <li>我是一个单选框</li> <li>我是一个单选框</li> <li>我是一个单选框</li> </ul>
<ul id="checkbox"> <li>我是一个多选框</li> <li>我是一个多选框</li> <li>我是一个多选框</li> <li>我是一个多选框</li> <li>我是一个多选框</li> <li>我是一个多选框</li> <li>我是一个多选框</li> </ul> </body> </html>
|
5.5 模拟下拉菜单
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90
| <!DOCTYPE html> <html lang="zh-cn"> <head> <meta charset="UTF-8"> <title>模拟下拉菜单</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; }
ul, li { list-style: none; }
.sel1 { width: 100px; margin: 100px auto 0px; }
.sel1 > .selBox { height: 27px; border: 1px solid #ccc; padding-left: 10px; line-height: 27px; background: url(../img/xiala.png) right center no-repeat; }
.sel1 > .selList { width: 100px; border: 1px solid #ccc; position: relative; top: -1px; display: none; }
.sel1 > .selList > li { height: 27px; line-height: 27px; padding-left: 10px; cursor: pointer; }
.sel1 > .selList > li:hover { background-color: lightgreen; }
.pink { background-color: pink; } </style> <script src="https://cdn.bootcss.com/jquery/1.12.4/jquery.min.js"></script> <script> $(function () { var text = $(".selBox").text(); for (let i = 0; i < $(".sel1 > .selList>li").length; i++) { if (text === $(".sel1>.selList >li").eq(i).text()) { $(".sel1>.selList> li").eq(i).addClass("pink"); } }
$(".selBox").click(function () { $(".selList").toggle(); }); $(".sel1>.selList>li").click(function () { $(".selBox").text($(this).text()); $(".selList").hide(); $(this).addClass("pink").siblings().removeClass("pink"); }); }); </script> </head> <body> <div class="sel1"> <div class="selBox">年付</div> <ul class="selList"> <li>月付</li> <li>季付</li> <li>年付</li> </ul> </div> </body> </html>
|
☆